PaymentSheetを使えば、1つのフローで決済を行うことができます。ユーザーが支払いボタンを押せば、すぐに支払いが完了します。(もし、この後に何らかのフローが必要な場合は、paymentFlow
メソッドを使用してください)。
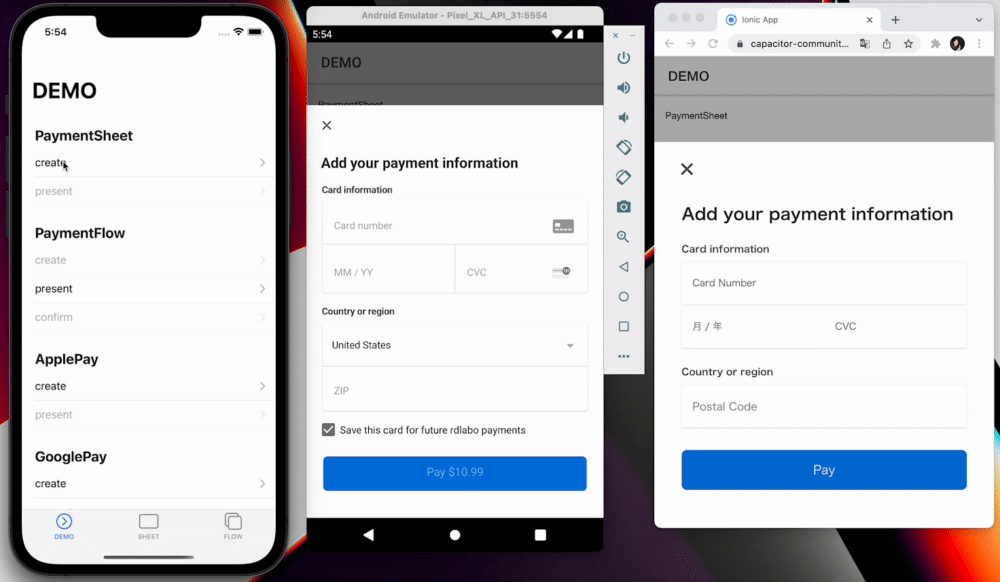
このメソッドは
PaymentIntent
による即時決済と SetupIntent
による将来の決済の両方に使用することができます。
これらのIntentが何なのかご存知ないですか?まずはStripeの公式サイトで学んでみてください。
PaymentIntent:
https://stripe.com/docs/payments/payment-intentshttps://stripe.com/docs/payments/payment-intents
SetupIntent:
https://stripe.com/docs/payments/save-and-reuse?platform=webhttps://stripe.com/docs/payments/save-and-reuse?platform=web
🐾 実装ガイド
1. createPaymentSheet
このメソッドはPaymentSheetのための設定です。使用する前に、バックエンドのエンドポイントに接続し、それぞれのキーを取得する必要があります。これは、このプラグインには「ない」機能です。そのため、
HTTPClient
、
Axios
、 Ajax
などを使用することになります。以下は、AngularのHttpClientの例です。この例では、paymentIntent
、ephemeralKey
、ephemeralKey
を取得しています。
Stripeはバックエンドの実装方法を提供しています:
https://stripe.com/docs/payments/accept-a-payment?platform=ios#add-server-endpointhttps://stripe.com/docs/payments/accept-a-payment?platform=ios#add-server-endpoint
その後、これらのキーを createPaymentSheet
メソッドで利用します。
import { Stripe, PaymentSheetEventsEnum } from '@capacitor-community/stripe';
(async () => {
const { paymentIntent, ephemeralKey, customer } = await this.http.post<{
paymentIntent: string;
ephemeralKey: string;
customer: string;
}>(environment.api + 'payment-sheet', {}).pipe(first()).toPromise(Promise);
await Stripe.createPaymentSheet({
paymentIntentClientSecret: paymentIntent,
customerId: customer,
customerEphemeralKeySecret: ephemeralKey,
});
})();
createPaymentSheet
は CreatePaymentSheetOption
のオプションを使用することができます。
method
createPaymentSheet(...)
createPaymentSheet(options: CreatePaymentSheetOption) => Promise<void>
プロパティ paymentIntentClientSecret
, customerId
, customerEphemeralKeySecret
は
必須 です。また、 デザインを設定して alwaysLight
か alwaysDark
にすることもできます。
ApplePay や GooglePay を PaymentSheetで設定することもできます。
interface
CreatePaymentSheetOption
Prop | Type |
Description | Default |
paymentIntentClientSecret |
string | Any documentation call 'paymentIntent' Set paymentIntentClientSecret or setupIntentClientSecret |
|
setupIntentClientSecret | string |
Any documentation call 'paymentIntent' Set paymentIntentClientSecret or setupIntentClientSecret |
|
billingDetailsCollectionConfiguration | BillingDetailsCollectionConfiguration |
Optional billingDetailsCollectionConfiguration | |
customerEphemeralKeySecret |
string | Any documentation call 'ephemeralKey' | |
customerId |
string | Any documentation call 'customer' | |
enableApplePay |
boolean | If you set payment method ApplePay, this set true | false |
applePayMerchantId | string | If set enableApplePay false, Plugin ignore here. |
|
enableGooglePay | boolean |
If you set payment method GooglePay, this set true | false |
GooglePayIsTesting |
boolean | | false, |
countryCode |
string | use ApplePay and GooglePay. If set enableApplePay and enableGooglePay false, Plugin ignore here. |
"US" |
merchantDisplayName | string |
| "App Name" |
returnURL |
string | | "" |
style |
'alwaysLight' | 'alwaysDark' | iOS Only | undefined |
withZipCode | boolean | Platform: Web only Show ZIP code field. |
true |
2. presentPaymentSheet
presentPaymentSheet
メソッドを実行すると、プラグインはPaymentSheetを提示し、結果を取得します。このメソッドは createPaymentSheet
の後に実行する必要があります。
(async () => {
const result = await Stripe.presentPaymentSheet();
if (result.paymentResult === PaymentSheetEventsEnum.Completed) {
}
})();
presentPaymentSheet
の返り値から PaymentSheetResultInterface
を取得することができます。
method
presentPaymentSheet()
presentPaymentSheet() => Promise<{ paymentResult: PaymentSheetResultInterface; }>
PaymentSheetResultInterface
は PaymentSheetEventsEnum
から作成されています。したがって、インポートして結果を確認する必要があります。
type alias
PaymentSheetResultInterface
PaymentSheetEventsEnum.Completed | PaymentSheetEventsEnum.Canceled | PaymentSheetEventsEnum.Failed
3. addListener
PaymentSheetのメソッドはリスナーを通知します。もし、支払い処理が完了したときのイベントを取得したい場合は、 Stripe
オブジェクトに
PaymentSheetEventsEnum.Completed
リスナーを追加する必要があります。
Stripe.addListener(PaymentSheetEventsEnum.Completed, () => {
console.log('PaymentSheetEventsEnum.Completed');
});
使用できるイベント名は
PaymentSheetEventsEnum
にあります。
enum
PaymentSheetEventsEnum
Members | Value |
Loaded |
"paymentSheetLoaded" |
FailedToLoad |
"paymentSheetFailedToLoad" |
Completed |
"paymentSheetCompleted" |
Canceled |
"paymentSheetCanceled" |
Failed |
"paymentSheetFailed" |
📖 Reference
詳しくはStripeのドキュメントをご覧ください。このプラグインはラッパーなので、詳しい情報はStripeのドキュメンテーションが役立ちます。
支払いを受け付ける(iOS)
このプラグインの PaymentSheet は pod 'Stripe'
を利用しています。
https://stripe.com/docs/payments/accept-a-payment?platform=ioshttps://stripe.com/docs/payments/accept-a-payment?platform=ios
支払いを受け付ける(Android)
このプラグインの PaymentSheet はcom.stripe:stripe-android
を利用しています。
https://stripe.com/docs/payments/accept-a-payment?platform=androidhttps://stripe.com/docs/payments/accept-a-payment?platform=android